출처: https://hybridclr.doc.code-philosophy.com/en/docs/beginner/quickstart
이 튜토리얼은 빈 프로젝트에서 HybridCLR 핫 업데이트를 경험하는 방법을 안내합니다. 단순화를 위해 빌드 대상이 Windows 또는 MacOS Standalone 플랫폼인 경우만 시연합니다.
독립 실행형 플랫폼에서 핫 업데이트 프로세스를 올바르게 실행한 다음 Android 및 iOS 플랫폼에서 핫 업데이트를 시도해 보세요. 프로세스는 매우 유사합니다.
경험 목표
- 핫 업데이트 어셈블리 만들기
- 핫 업데이트 어셈블리를 로드하고 핫 업데이트 코드를 실행하여 Hello, HybridCLR를 인쇄합니다.
- 핫 업데이트 코드를 수정하여 Hello, World를 출력합니다.
환경 준비
Unity 설치
CAUTION
HybridCLR은 Unity 2019~2022의 모든 LTS 버전을 지원합니다. 현재 사용 중인 버전이 아래 권장 버전에 포함되지 않는 경우 Install HybridCLR 문서를 참조하세요.
- 2019.4.40, 2020.3.26+, 2021.3.0+, 2022.3.0+ 버전 설치
- 운영 체제에 따라 설치 중 모듈을 선택할 때 Windows 빌드 지원(IL2CPP) 또는 Mac 빌드 지원(IL2CPP)를 선택해야 합니다.
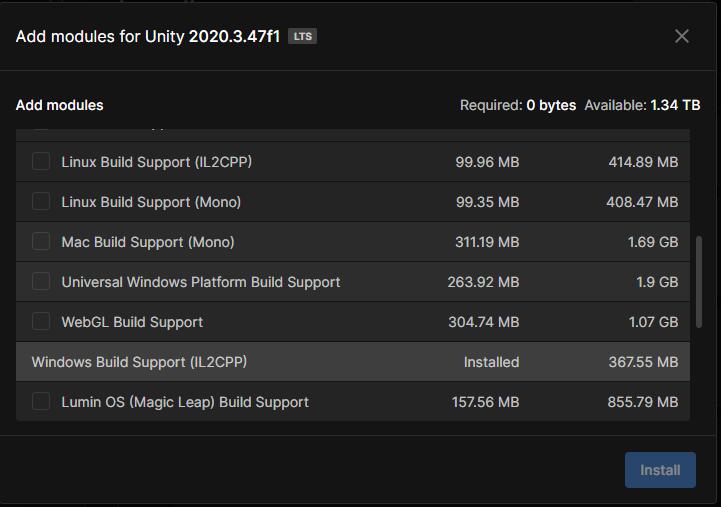
IDE 및 관련 컴파일 환경 설치
- Win에서는 visual studio 2019 이상을 설치해야 합니다. 설치 시 최소한 유니티로 게임 개발 및 C++로 게임 개발 구성 요소를 포함해야 합니다.
- install git
- MacOS 버전 = 12, xcode 버전 = 13이 필요합니다(예: xcode 13.4.1, macos 12.4.
- install git
- install cmake
Unity 핫 업데이트 프로젝트 초기화
핫 업데이트 프로젝트를 처음부터 빌드하는 과정은 지루합니다. 프로젝트 구조, 리소스 및 코드는 hybridclr_trial 프로젝트를 참조할 수 있으며, 해당 저장소 주소는 github 또는 gitee입니다.
프로젝트 생성
빈 Unity 프로젝트를 생성합니다.
ConsoleToScreen.cs 스크립트를 생성합니다.
이 스크립트는 핫 업데이트 시연에 직접적인 영향을 미치지 않습니다. 로그를 화면에 인쇄할 수 있으므로 오류를 찾는 데 편리합니다.
Assets/ConsoleToScreen.cs 스크립트 클래스를 생성하며, 코드는 다음과 같습니다:
using System; using System. Collections; using System.Collections.Generic; using UnityEngine;
public class ConsoleToScreen : MonoBehaviour { const int maxLines = 50; const int maxLineLength = 120; private string _logStr = "";
private readonly List<string>_lines = new List<string>();
public int fontSize = 15;
void OnEnable() { Application. logMessageReceived += Log; } void OnDisable() { Application. logMessageReceived -= Log; }
public void Log(string logString, string stackTrace, LogType type) { foreach (var line in logString. Split('\n')) { if (line. Length <= maxLineLength) { _lines. Add(line); continue; } var lineCount = line.Length / maxLineLength + 1; for (int i = 0; i < lineCount; i++) { if ((i + 1) * maxLineLength <= line.Length) { _lines.Add(line.Substring(i * maxLineLength, maxLineLength)); } else { _lines.Add(line.Substring(i * maxLineLength, line.Length - i * maxLineLength)); } } } if (_lines. Count > maxLines) { _lines. RemoveRange(0, _lines. Count - maxLines); } _logStr = string. Join("\n", _lines); }
void OnGUI() { GUI.matrix = Matrix4x4.TRS(Vector3.zero, Quaternion.identity, new Vector3(Screen. width / 1200.0f, Screen. height / 800.0f, 1.0f)); GUI.Label(new Rect(10, 10, 800, 370), _logStr, new GUIStyle() { fontSize = Math.Max(10, fontSize) }); } } |
기본 장면 만들기
- 기본 초기 씬 main.scene 생성
- 씬에 빈 게임 오브젝트를 생성하고 그 위에 콘솔투스크린을 걸어 놓습니다.
- 빌드 설정에서 패키지 씬 목록에 메인 씬을 추가합니다.
핫업데이트 핫 업데이트 모듈 생성
- 자산/핫업데이트 디렉토리 만들기
- 디렉토리에서 생성/어셈블리 정의를 마우스 오른쪽 버튼으로 클릭하여 HotUpdate라는 이름의 어셈블리 모듈을 생성합니다.
HybridCLR 설치 및 구성하기
com.code-philosophy.hybridclr 패키지를 설치합니다.
메인 메뉴에서 Windows/패키지 관리자를 클릭하여 패키지 관리자를 엽니다. 아래와 같이 Add package from git URL...를 클릭하고 https://gitee.com/focus-creative-games/hybridclr_unity.git 또는 https://github.com/focus-creative -games/hybridclr_unity.git을 입력합니다.
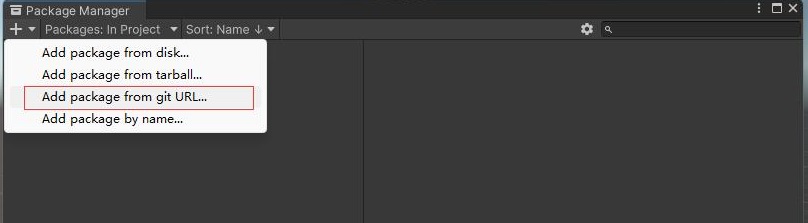
URL에서 패키지를 설치하는 데 익숙하지 않은 경우 giturl에서 설치하기를 참조하세요.
국내 네트워크 사정으로 인해 Unity에서 네트워크 예외가 발생하여 설치에 실패할 수 있습니다. 먼저 com.code-philosophy.hybridclr를 로컬에 복제 또는 다운로드하고 폴더 이름을 com.code-philosophy.hybridclr로 변경한 후 프로젝트의 Packages 디렉토리로 직접 이동합니다.
com.code-philosophy.hybridclr를 초기화합니다.
하이브리드CLR/인스톨러... 메뉴를 열고 설치 버튼을 클릭하여 설치합니다. 약 30초간 기다립니다. 설치가 완료되면 마지막에 설치 성공 로그가 인쇄됩니다.
HybridCLR 구성
HybridCLR/Settings 메뉴를 열고 아래와 같이 HotUpdate 어셈블리를 Hot Update Assemblies 구성 항목에 추가합니다:
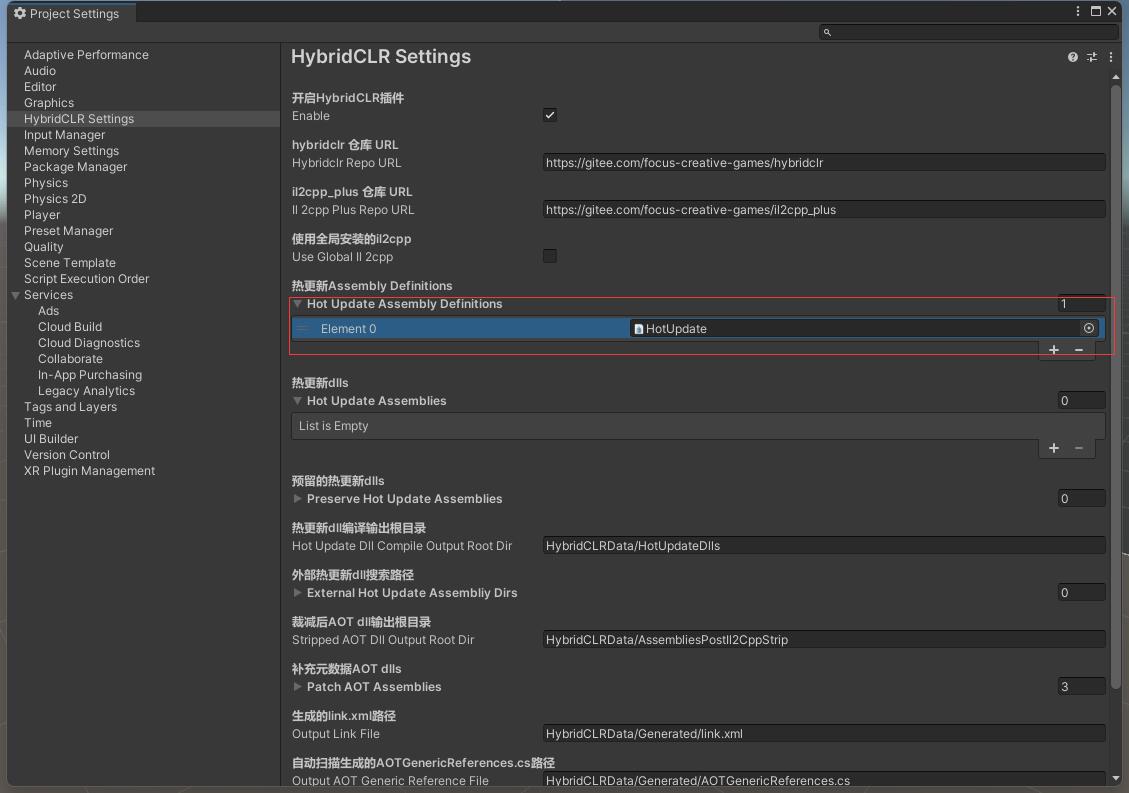
Configure PlayerSettings
- if your package version less than v4.0.0, you have to turn off the incremental GC (Use Incremental GC) option. Because incremental GC is not currently supported.
- Scripting Backend switched to IL2CPP.
- Api Compatability Level switched to .Net 4.x (Unity 2019-2020) or .Net Framework (Unity 2021+).
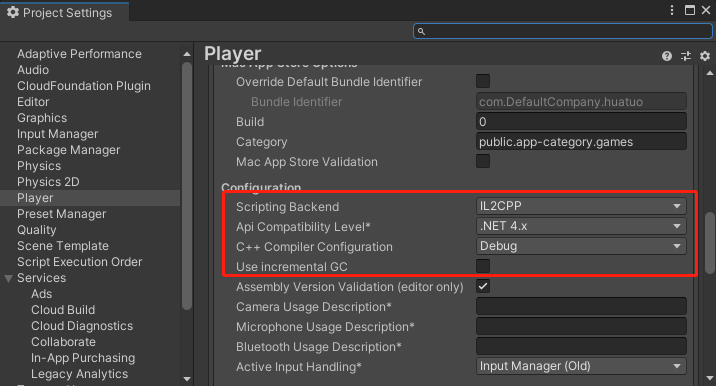
Create hot update script
Create Assets/HotUpdate/Hello.cs file, the code content is as follows
using System. Collections; using UnityEngine;
public class Hello { public static void Run() { Debug. Log("Hello, HybridCLR"); } } |
핫 업데이트 부분의 코드가 다른 솔루션처럼 C# 구문에 제한이 있는 것은 아닌지 걱정하실 수 있습니다. HybridCLR은 거의 완벽하게 구현되어 있으며 핫 업데이트 코드에 대한 제한이 거의 없으므로 직접 작성해 보시기 바랍니다.
드문 예외에 대해서는 지원되지 않는 기능를 참조하세요.
핫 업데이트 어셈블리 로드
데모를 단순화하기 위해 http 서버를 통해 핫업데이트.dll을 다운로드하지 않고 스트리밍 자산 디렉토리에 핫업데이트.dll을 직접 넣습니다.
HybridCLR은 네이티브 런타임 구현이므로 Assembly Assembly.Load(byte[])를 호출하여 핫 업데이트 어셈블리를 로드합니다.
Assets/LoadDll.cs 스크립트를 생성한 다음 기본 장면에 게임 오브젝트를 생성하고 LoadDll 스크립트를 마운트합니다.
using HybridCLR; using System; using System. Collections; using System.Collections.Generic; using System.IO; using System. Linq; using System. Reflection; using System. Threading. Tasks; using UnityEngine; using UnityEngine. Networking; public class LoadDll : MonoBehaviour {
void Start() { // In the Editor environment, HotUpdate.dll.bytes has been automatically loaded and does not need to be loaded. Repeated loading will cause problems. #if !UNITY_EDITOR Assembly hotUpdateAss = Assembly.Load(File.ReadAllBytes($"{Application.streamingAssetsPath}/HotUpdate.dll.bytes")); #else // No need to load under Editor, directly find the HotUpdate assembly Assembly hotUpdateAss = System.AppDomain.CurrentDomain.GetAssemblies().First(a => a.GetName().Name == "HotUpdate"); #endif } } |
핫 업데이트 코드 호출
물론 메인 프로젝트는 핫 업데이트 코드를 직접 참조할 수 없습니다. 메인 프로젝트에서 핫 업데이트 어셈블리의 코드를 호출하는 방법에는 여러 가지가 있습니다. 여기서는 리플렉션을 통해 핫 업데이트 코드를 호출합니다.
LoadDll.Start 함수 뒤에 리플렉션 호출 코드를 추가하면 다음과 같이 최종 코드가 완성됩니다:
void Start() { // In the Editor environment, HotUpdate.dll.bytes has been automatically loaded and does not need to be loaded. Repeated loading will cause problems. #if !UNITY_EDITOR Assembly hotUpdateAss = Assembly.Load(File.ReadAllBytes($"{Application.streamingAssetsPath}/HotUpdate.dll.bytes")); #else // No need to load under Editor, directly find the HotUpdate assembly Assembly hotUpdateAss = System.AppDomain.CurrentDomain.GetAssemblies().First(a => a.GetName().Name == "HotUpdate"); #endif Type type = hotUpdateAss. GetType("Hello"); type. GetMethod("Run"). Invoke(null, null); } |
지금까지 전체 핫 업데이트 프로젝트의 생성이 완료되었습니다! ! !
에디터에서 체험판 실행
메인 장면을 실행하면 코드가 정상적으로 작동하고 있음을 나타내는 'Hello, HybridCLR'이 화면에 표시됩니다.
패키징 및 실행
- HybridCLR/Generate/All 메뉴를 실행하여 필요한 생성 작업을 수행합니다. 이 단계는 놓칠 수 없습니다!!!
- {proj}/HybridCLRData/HotUpdateDlls/StandaloneWindows64(MacOS에서는 StandaloneMacXxx) 디렉터리에 있는 HotUpdate.dll을 Assets/StreamingAssets/HotUpdate에 복사하세요.dll.bytes, 주, .bytes 접미사를 추가해야 합니다! ! !
- 빌드 설정 대화 상자를 열고 빌드 및 실행를 클릭한 후 핫 업데이트 샘플 프로젝트를 패키징하고 실행합니다.
패키징에 성공하고 화면에 'Hello, HybridCLR'이 표시되면 핫 업데이트 코드가 성공적으로 실행된 것입니다!
핫 업데이트 테스트
- 자산/핫업데이트/헬로.cs의 실행 함수에서 Debug.Log("Hello, HybridCLR"); 코드를 Debug.Log("Hello, World");로 수정합니다.
- 메뉴 명령 HybridCLR/CompileDll/ActiveBulidTarget를 실행하여 핫 업데이트 코드를 다시 컴파일합니다.
- {proj}/HybridCLRData/HotUpdateDlls/StandaloneWindows64(MacOS에서는 StandaloneMacXxx) 디렉터리에 있는 HotUpdate.dll을 방금 패키지 출력 디렉터리의 XXX_Data/StreamingAssets/HotUpdate.dll.bytes에 복사하세요.
- 프로그램을 다시 실행하면 화면에 핫 업데이트 코드가 적용되었음을 나타내는 Hello, World가 표시됩니다!
이것으로 핫 업데이트 체험이 완료되었습니다! ! !